3 Simple Tricks to Make Your Laravel Code Less Messy
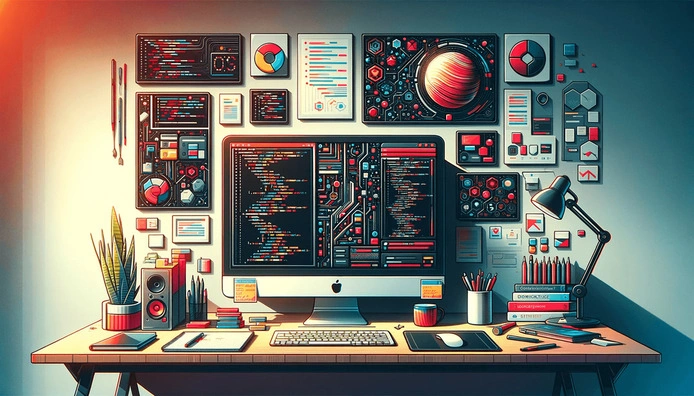
Introduction
Laravel is a popular PHP framework that provides clean and elegant syntax, making it easy to write high-quality web applications. However, most beginners tend to overlook a lot of powerful features offered by the framework. In this article, we will explore three simple tricks that can help you achieve this: form request validation, Eloquent's fill method, and the use of route bindings. These techniques will help you write cleaner, more maintainable code while making your Laravel applications more efficient.
Form Request Validation
Form request validation is a powerful way to handle data validation in Laravel. It separates validation logic from the controller, making your code more organized and maintainable. With form requests, you can create custom request classes that include validation rules, messages, and even authorization logic.
For instance, let’s consider the following code written in the TransactionController
that creates a new instance of the Transaction
model and stores it in the database:
The validation part of the code clearly doesn’t belong in the store
method as controllers should be focused on their primary responsibility, which is handling HTTP requests and responses. On top of that, you might need a similar validation logic somewhere else so it would be better if it was separated from the rest of the logic. Let’s use form requests for that reason.
First, we create a custom form request class using the make:request
command:
Next, we can transfer the validation rules inside the rules()
method:
Finally, type-hint the custom request class in our controller method, and Laravel will automatically validate the incoming request:
Eloquent's fill Method
The Eloquent ORM (Object-Relational Mapping) in Laravel simplifies database interactions and makes it easy to work with your application's data. The fill()
method allows you to set multiple attributes on a model at once while respecting the model's $fillable
property. In our previous example, instead of assigning the attributes manually, we could use the fill()
method instead. Let’s define the $fillable
property in our Eloquent model that will contain all the fields we want to assign:
Now, we can use the fill()
method to update the model's attributes:
Using the fill()
method ensures that only the attributes specified in the $fillable
property are updated, improving security and reducing the chances of accidental data manipulation.
Route Model Binding
Another technique that contributes to cleaner code in Laravel is Route Model Binding, which enables you to automatically inject model instances into your route or controller methods. Route Model Binding simplifies your code by reducing the need for repetitive boilerplate code to fetch data from the database.
Implicit Route Model Binding
Using the Transaction model as an example, let's assume it has the following fields: amount
, description
, and date
. Laravel can automatically resolve Eloquent models in your routes or controller methods. This is called Implicit Route Model Binding.
Example:
Consider the following route definition in routes/web.php
:
Now, you can type-hint the Eloquent model in your controller method, and Laravel will automatically fetch the corresponding model instance:
Laravel retrieves the Transaction model instance based on the {transaction}
route parameter and passes it to the show method. If the model instance is not found, Laravel generates a 404 response.
Conclusion
Adopting best practices and utilizing Laravel's powerful features can significantly improve the organization, readability, and maintainability of your code. In this article, we discussed how form request validation, Eloquent's fill method, and Route Model Binding contribute to cleaner code in your Laravel applications. Additionally, we demonstrated how to adapt a traditional web route and controller method for use as an API endpoint, further expanding the versatility of the Laravel framework. By implementing these techniques in your projects, you can create efficient, maintainable, and secure web applications that are easy to understand and scale as needed. Happy coding!